Mesh Renderer
CMU 15-662 Computer Graphics Course
A mesh renderer built during the Computer Graphics course (Spring 2024) at Carnegie Mellon Univeristy. Used the skeleton code provided by the course at https://github.com/CMU-Graphics/Scotty3D.
Scotty3D is a 3D graphics suite where I implemented mesh editing, rasterization, path tracing, animation interpolation, and physics simulation.
Rasterizer: Implemented transformations, triangle rendering, depth blending, interpolation, mip-mapping for texture anti-aliasing, and supersampling
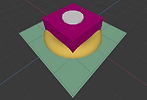
Before
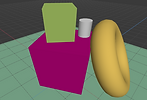
After
Transforms
Implemented scene graph transformations by completing local_to_world() and world_to_local(), enabling hierarchical object positioning through scaling, rotation, and translation in world space.
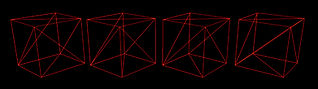
Line, Triangle Rasterization & Depth Testing
Line rasterization - Bresenham's Algorithm and diamond-exit rule.
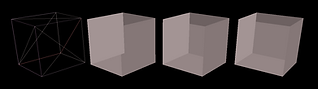
Triangle rasterization - Standard Algorithm and top-left rule for intersection.

Alpha blending and depth testing for correct pixel composition
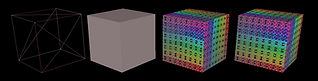
Triangle Interpolation
Interpolated triangle vertices' positions using barycentric coordinates. Screen-space smooth triangles (3rd cube) and perspective-correct triangles (4th cube)
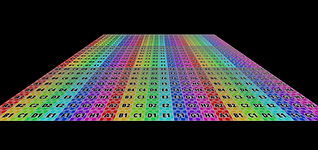
Mip-Mapping for texture anti-aliasing and sampling
Nearest, bilinear and trilinear sampling. Ordered levels from high-res to low-res along the z-axis.

Before

After
Supersampling
Implemented a framebuffer for subpixel sampling and weighted color averaging to enhance anti-aliasing, adjusted clipped vertex positions for smoother edges, and applied texture mapping with bilinear filtering
Path Tracer: Implemented camera ray generation, ray-primitive intersection, and BVH acceleration for efficient ray traversal, along with path tracing, material shading, and direct and environment lighting
Final Outcome

Materials: Mirror - perfect specular reflection (red shoe holder / table top) Refract - Snell's Law, BSDF attenuation using physical based rendering (shoe on the left) Glass - Schlick's approximation. Reflect + refract. (shoe on the right, blue shoe holder / table top) Lighting: Direct lighting - reduce variance by sampling light source directly. single-sample multiple importance sampling Lambertian BSDF and indirect lighting - simulate the complicated paths that light can take throughout the scene, bouncing off many surfaces before eventually reaching the camera (small shoe on the right) Environment lighting - uniformly sampling, then importance sampling the environment map (CDF and PDF) with Jacobian (room background)
Step by Step Learnings & Builds
Camera Rays
Implemented camera ray generation by transforming pixel coordinates into world-space rays using field of view, aspect ratio, and sensor size.
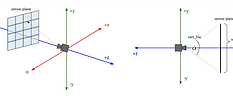

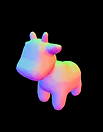
Intersecting Objects
Used Möller-Trumbore algorithm and Cramer’s rule for triangle intersection. The shading normal for a triangle is computed by linear interpolation from per-vertex normals using the barycentric coordinates of the hit point as their weights.
Bounding Volume Hierchy (BVH)
1. Bounding Box Calculation & Intersection
2. Surface Area Heuristic for BVH Construction
3. Ray-BVH Intersection using recursion

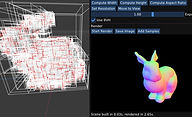
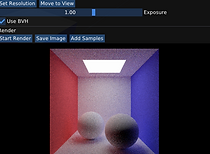
Global Illumination & Path Tracing
Implemented Lambertian BSDF, indirect and direct lighting estimation, and next-event estimation by splitting samples between BSDF scattering and area lights. Integrated mixture sampling and multiple-importance sampling to reduce variance, leveraging Monte Carlo estimation for accurate global illumination.
Materials & Specular Reflection/Refraction
Implemented mirror and glass materials using perfect specular reflection (via vector reflection) and refraction (via Snell’s Law), handling total internal reflection when necessary. Used Fresnel equations (or Schlick’s approximation) to determine reflection vs. transmission ratios for dielectric materials, enabling realistic light interactions through multiple-importance sampling in path tracing.
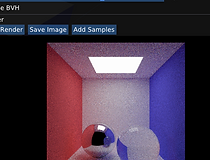
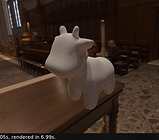
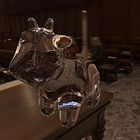
Lambert
Glass
Environment Lighting & Importance Sampling
Implemented environment lighting using HDR environment maps, first with uniform sampling and then with importance sampling to reduce variance. Built a probability distribution function (PDF) and cumulative distribution function (CDF) for efficient inversion sampling, accounting for pixel solid angles and brightness. Applied Jacobian correction to properly map environment map pixels to the unit sphere for accurate light sampling.
Fun Debuggings Before Success

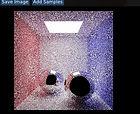
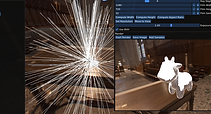
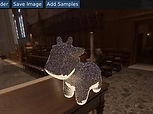
Mesh Editing: Implemented half-edge mesh data structures, edge flip and split, face extrusion, triangulation, linear subdivision, Catmull-Clark subdivision, Isotropic Remeshing.
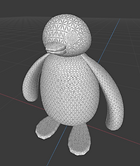
Before
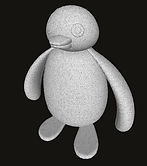
After
Mesh Edit Outcome
Triangulation, subdivision, and remeshing.
Concepts
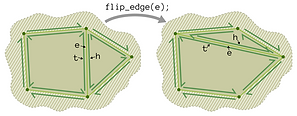
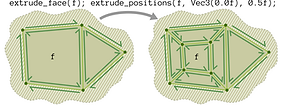
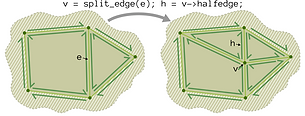
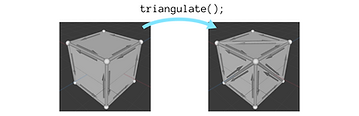
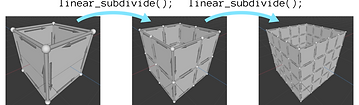
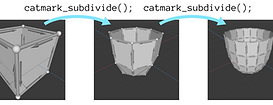
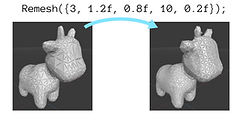
Animation: Skeletal Motion & Particle Simulation
Implemented spline interpolation for smooth keyframe transitions, skeletal kinematics for joint transformations, and linear-blend skinning for realistic mesh deformation. Added particle simulation to model dynamic motion, integrating forces and collisions for natural behavior.
Final Outcome
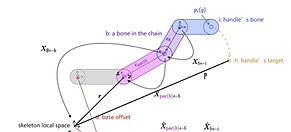
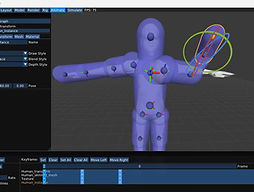